
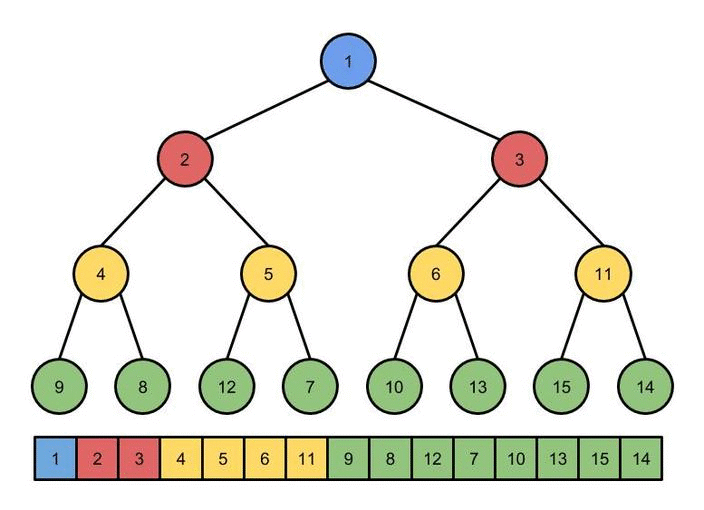
(i.e., the array has more elements than the queue), the element in If the queue fits in the specified array with room to spare Specified array and the size of this queue. Otherwise, a new array is allocated with the runtime type of the If the queue fits in the specified array, it is returned therein. The returned array elements are in no particular order. Runtime type of the returned array is that of the specified array. Returns an array containing all of the elements in this queue the Methods and constant time for the retrieval methods Linear time for the remove(Object) and contains(Object) O(log(n)) time for the enqueuing and dequeuing methods Implementation note: this implementation provides Instead, use the thread-safe PriorityBlockingQueue class. Instance concurrently if any of the threads modifies the queue. Multiple threads should not access a PriorityQueue Note that this implementation is not synchronized. Traversal, consider using Arrays.sort(pq.toArray()). The priority queue in any particular order. The Iterator provided in method iterator() is not guaranteed to traverse the elements of Optional methods of the Collection and Iterator interfaces. This class and its iterator implement all of the
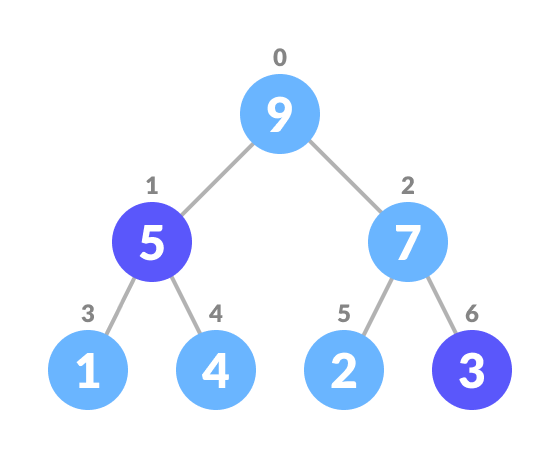
As elements are added to a priority queue, its capacity It is always at least as large as the queue The queue retrieval operations poll,Ī priority queue is unbounded, but has an internalĬapacity governing the size of an array used to store theĮlements on the queue. Tied for least value, the head is one of those elements - ties areīroken arbitrarily. The head of this queue is the least element Insertion of non-comparable objects (doing so may result in A priority queue does not permit null elements.Ī priority queue relying on natural ordering also does not permit Provided at queue construction time, depending on which constructor is The elements of the priority queue are ordered according to their The time complexity is O(logn).An unbounded priority queue based on a priority heap. We call this process bubble up or heap up. If it’s value larger than its parent, it should be switched the position with its parent. Then we move it up based on its value compared to its parent. To enqueue, we put the new value at the end of the array. length is actual number of elements in the array. We declare three attributes: heap, length and maxSize. Part 4 – priority queue implementation with heap – recursive solution Part 3 – priority queue implementation with heap – iterative solution Part 2 – priority queue implementation with unordered array Part 1 – priority queue implementation with ordered array If we want ascending priority, we can use min heap to implement. Please note when we use max heap, the priority queue is a descending priority (ie the bigger the key, the higher the priority). Based on this, we can use heap to implement priority queue. If we read the value level by level from top to bottom, all elements are partially sorted. Meanwhile, heap should also meets this criteria: the parent’s key is larger than both children’s keys. The relationship between the nodes in the heap has following formulas: Each node’s position in the tree is corresponding to the index in the array as following diagram. Based on these characteristic, it can be represented as an array. Heap is a complete binary tree, A complete binary tree is a binary tree in which all levels are completely filled and all the nodes in the last level are as left as possible. The element with the highest priority shall be dequeued first. A priority queue is a queue in which we insert an element at the back (enqueue) and remove an element from the front (dequeue).
